In this hands-on guide, we’ll dive into setting up Continuous Deployment (CD) using AWS CodeDeploy, a powerful managed service that automates application deployments. Before we begin, it’s important to complete the Continuous Integration (CI) phase using AWS services such as CodeBuild, CodePipeline, and GitHub. If you haven’t set up CI yet, I’ve covered this essential step in a previous project, which you can find [here].
Now, let’s streamline the deployment process using AWS CodeDeploy to ensure a smooth transition from CI to CD.
For more of my projects, please visit my website here
Tools and Technologies Used
AWS CodePipeline: A continuous integration and delivery service that orchestrates the release process.
AWS CodeDeploy: A managed deployment service that automates software deployments to various computing services, including Amazon EC2 instances, AWS Lambda functions, and on-premises servers.
Amazon EC2:Scalable virtual servers in the cloud, which serve as deployment targets for AWS CodeDeploy.
These tools enable efficient automation and scalability, ensuring reliable and consistent application releases without the need to manage underlying infrastructure.
Create an EC2 Instance
While this process can be automated using tools like Terraform, we’ll walk through the manual steps for simplicity.
Here’s a detailed step-by-step guide to creating an EC2 instance:
Log into the AWS Management Console.
Navigate to the EC2 Dashboard: In the search bar at the top, type EC2.
Under Services, select EC2 to access the EC2 dashboard.
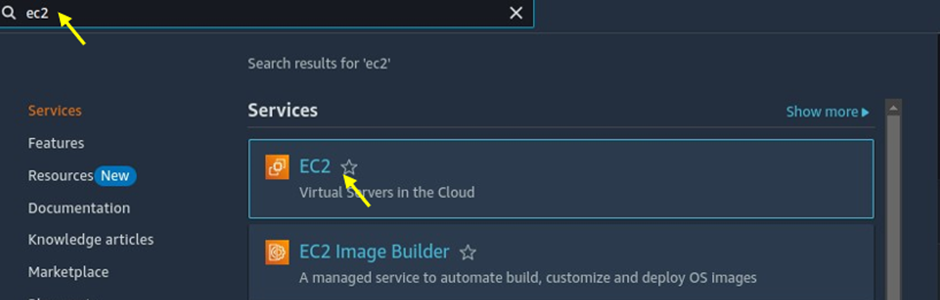
On the left-hand side of the EC2 dashboard, click on Instances then in the top-right corner, click Launch Instance.
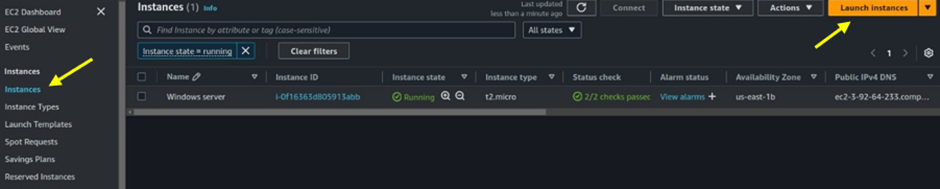
Fill in your Instance details, under application and OS images, select the QuickStart tab then select Ubuntu.
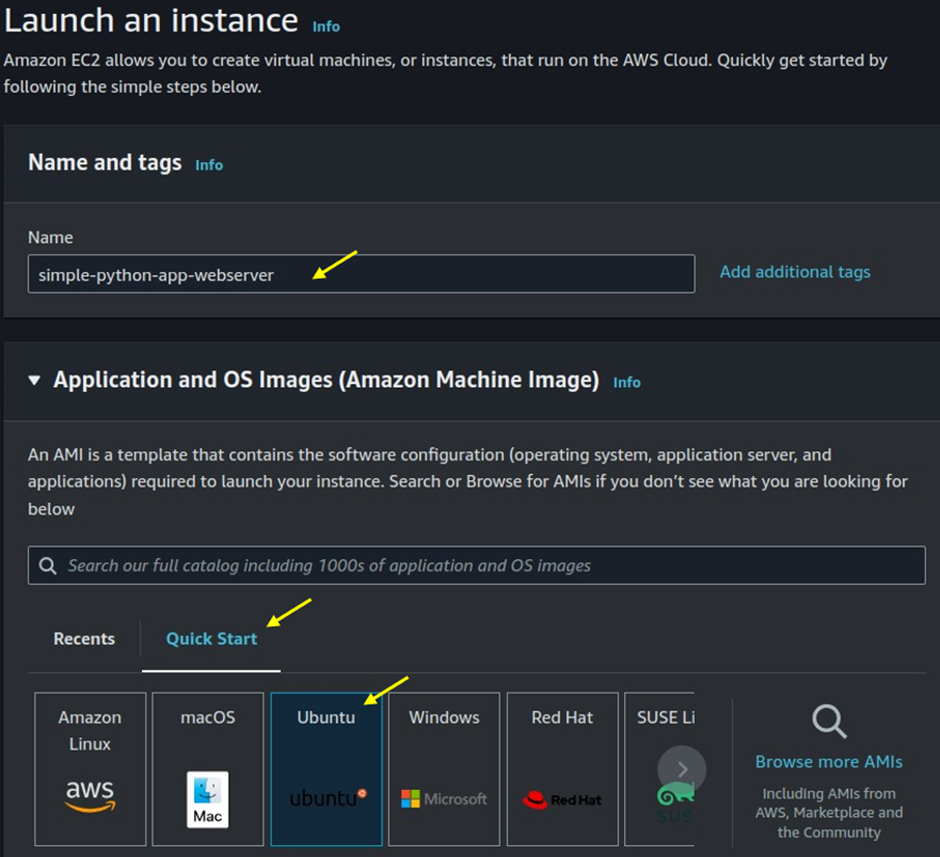
For Instance, type, select t2. Micro which is free tier eligible.
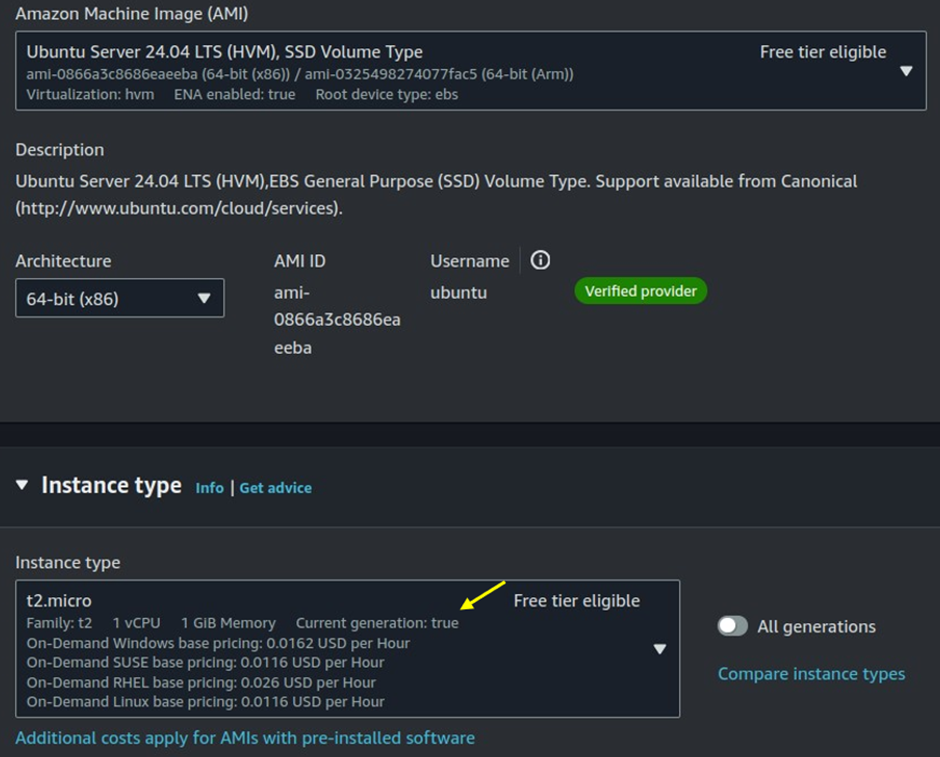
Under the key-pair name, select the drop-down button then select your key-pair.
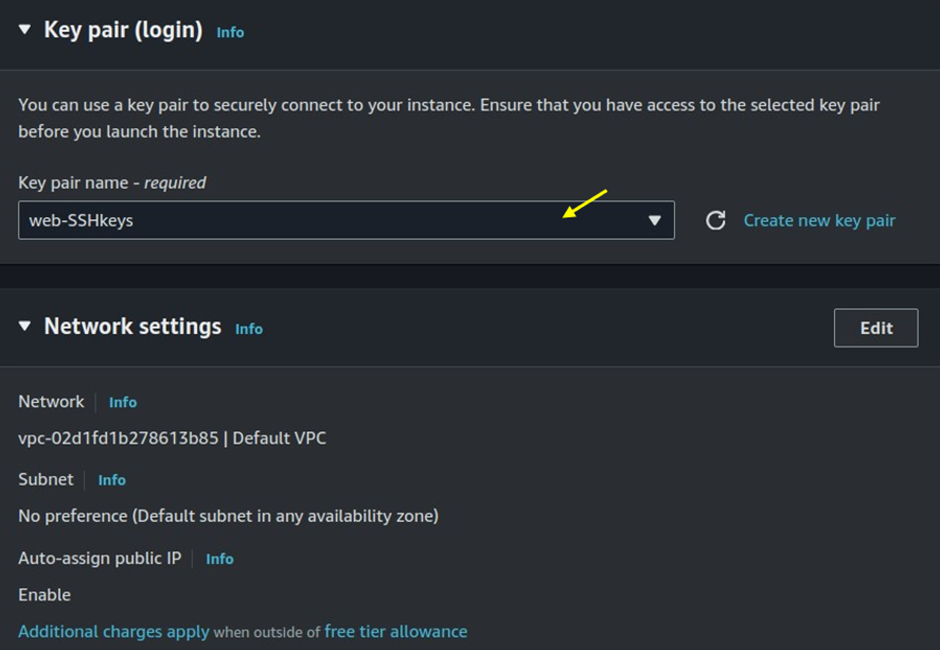
Leave the Networking settings as default.
Under Firewall (Security Groups), select Create a new security group then click to allow SSH, HTTPS, and HTTP traffic.
As a best practice, ensure port 22 (SSH)is not open to everyone. Restrict access by specifying trusted IP addresses or ranges.
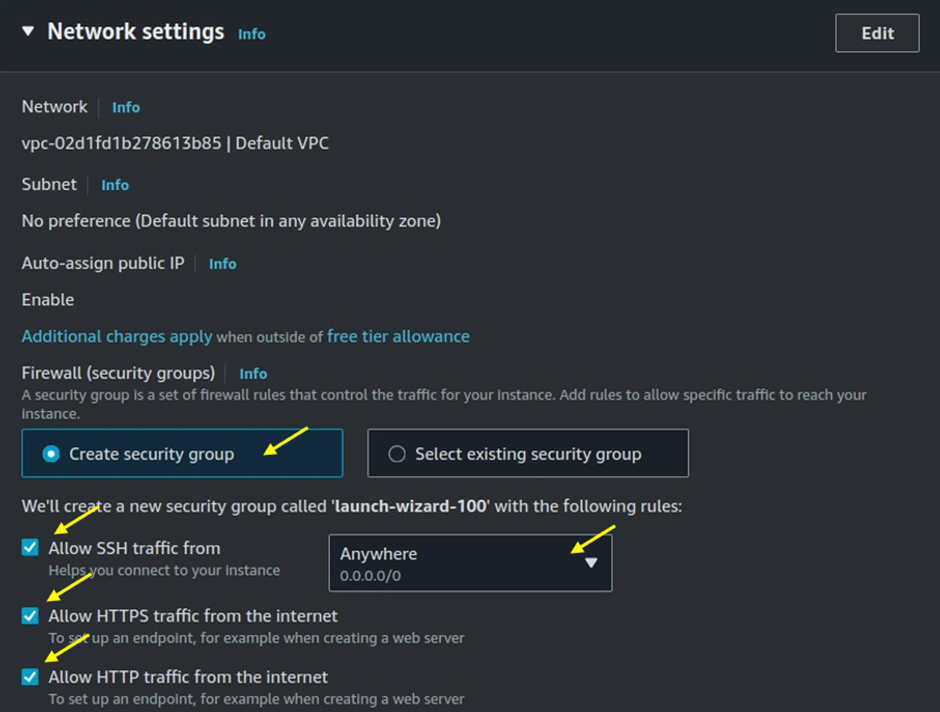
For this demo, the default 8GB storage is sufficient, so leave the storage settings as default.
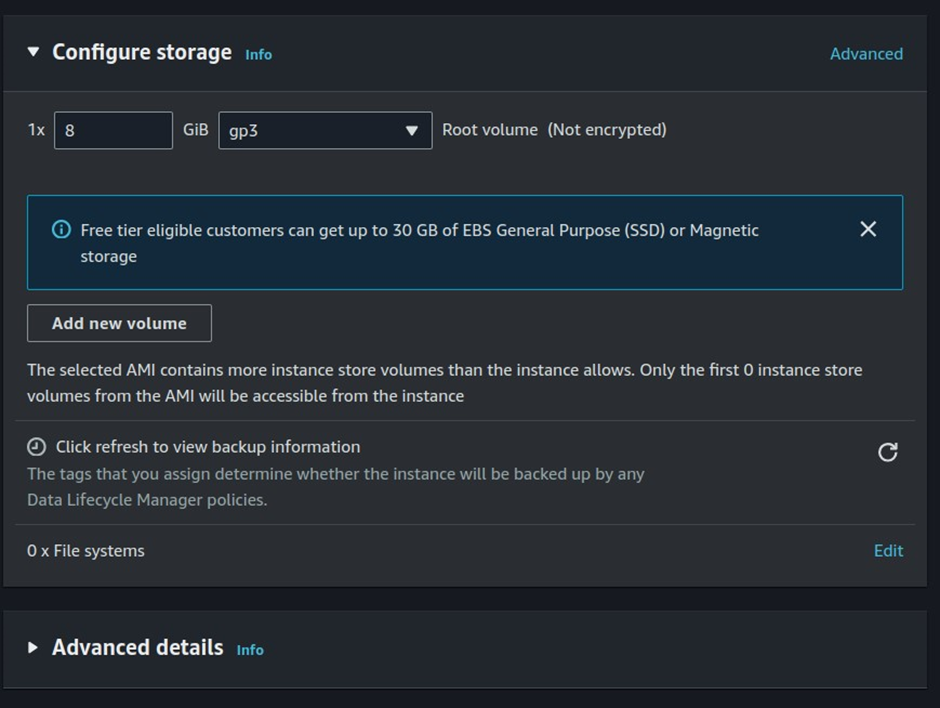
Review all the instance details to ensure everything is configured correctly and once satisfied, click Launch Instance.
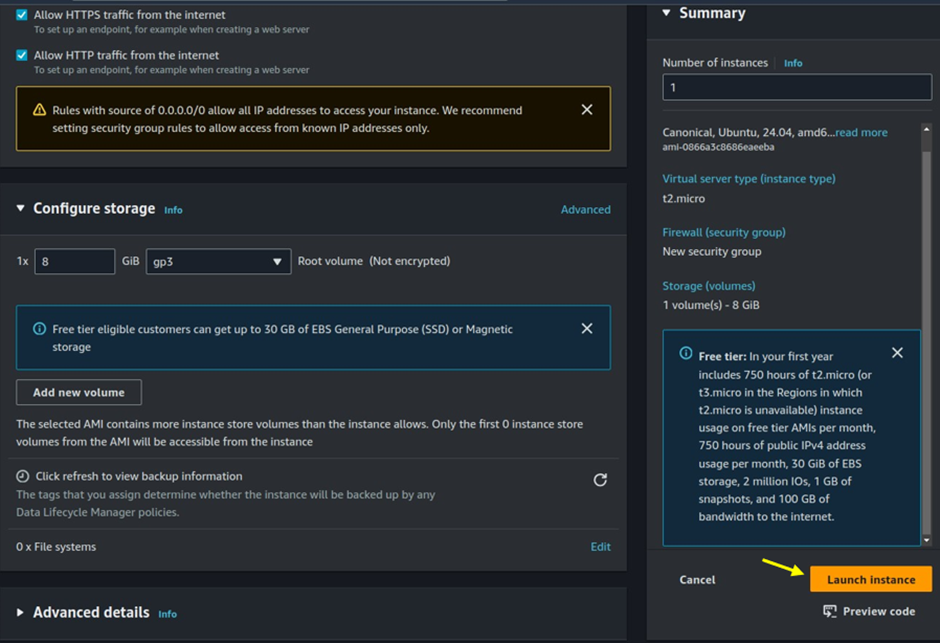
Add Tags: Once your instance is up and running, navigate to the Instance Dashboard.
Click on Manage Tags, then add tags to your instance as needed (e.g., “Name: simple-python-app-webserver”).
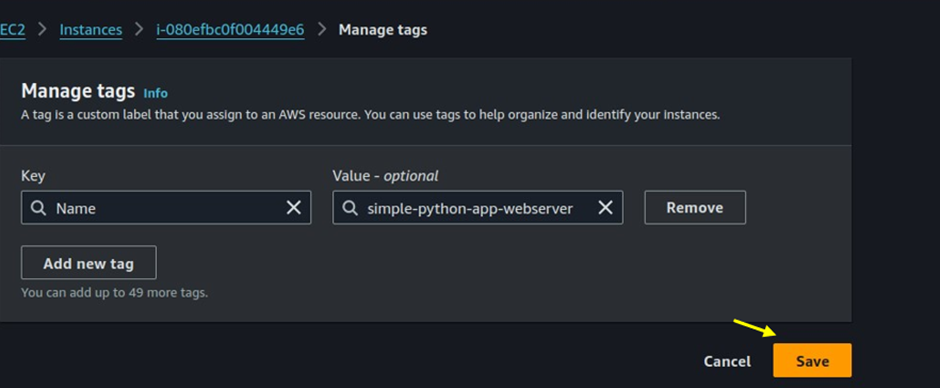
Create and Attach an IAM Role: To allow your EC2 instance to communicate with the CodeDeploy service, navigate to the IAM Dashboard in the AWS Management Console.
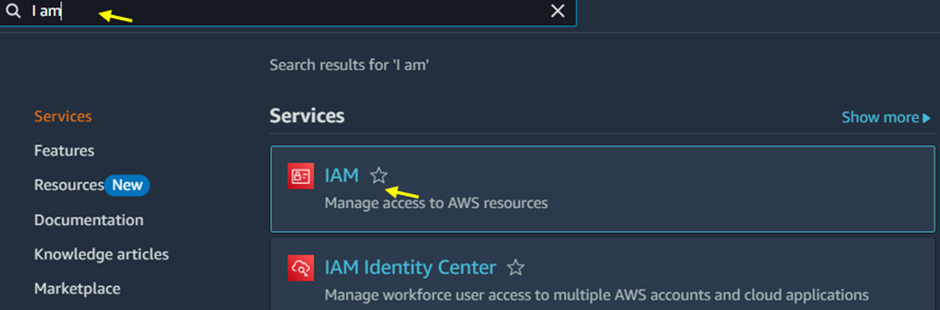
In the IAM Dashboard, click on Roles. Then, click Create Role.
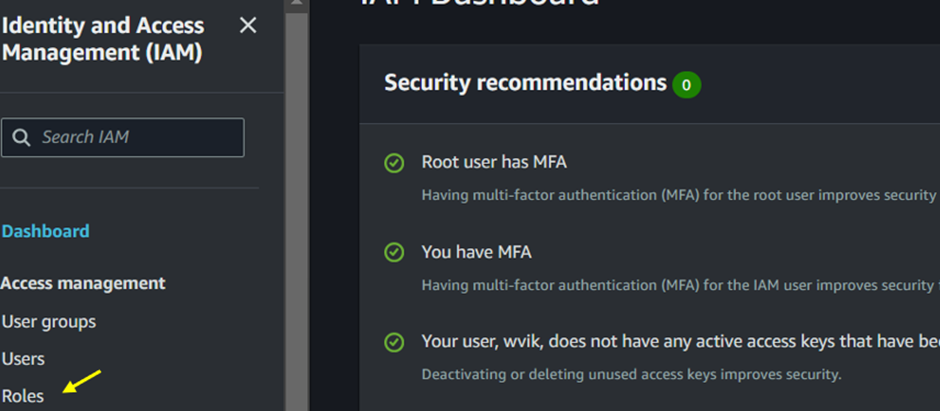
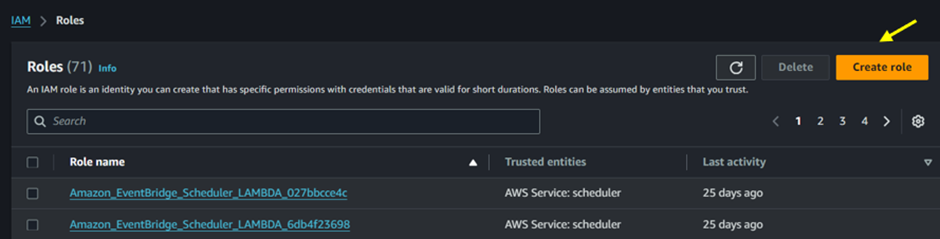
Choose AWS Service as the trusted entity then select CodeDeploy from the list of services.
Click Next to proceed.
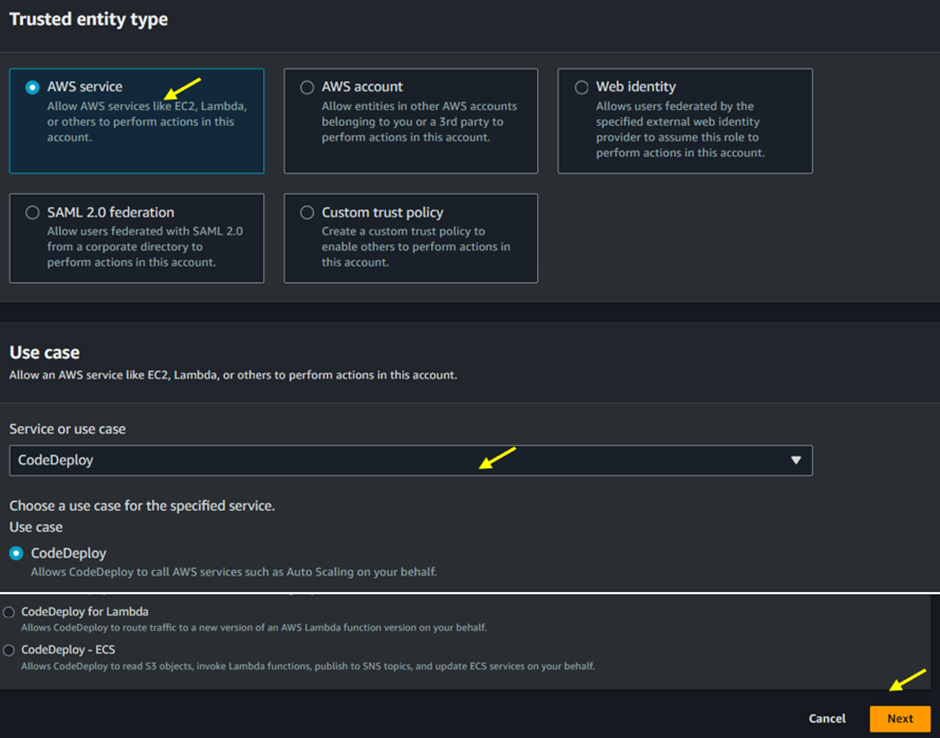
Click next again.
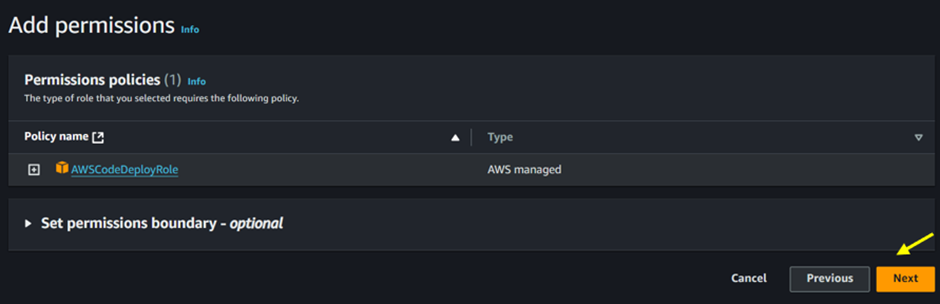
Enter a name for your role. Scroll down and click Create Role.
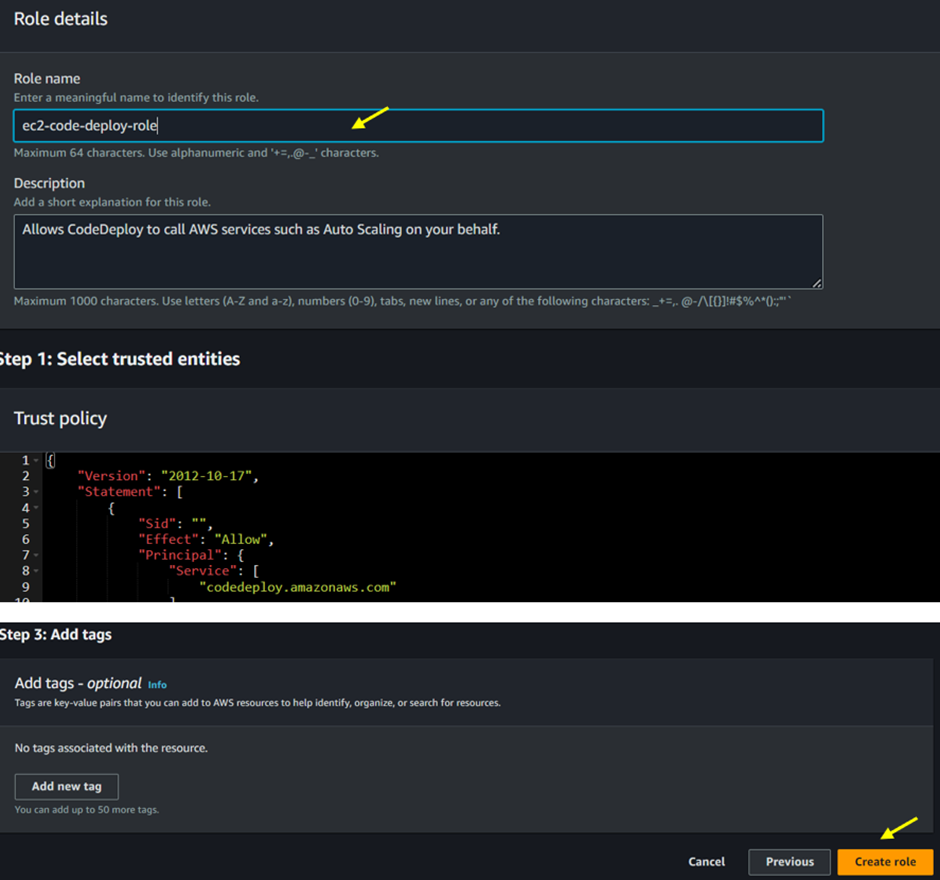
Lets add Permissions to the Role:
To add permissions to your newly created role, navigate to the Permissions tab associated with the role then click on Attach Policies to grant the necessary permissions.
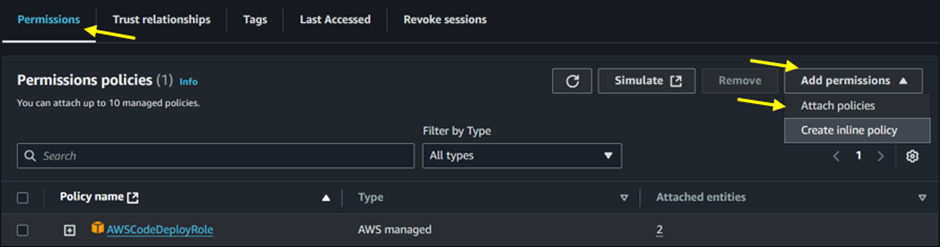
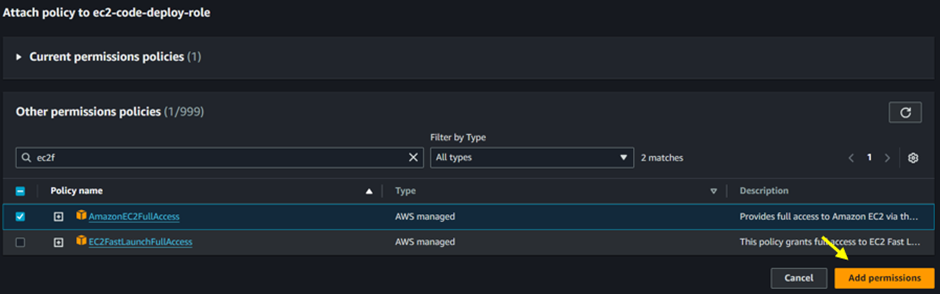
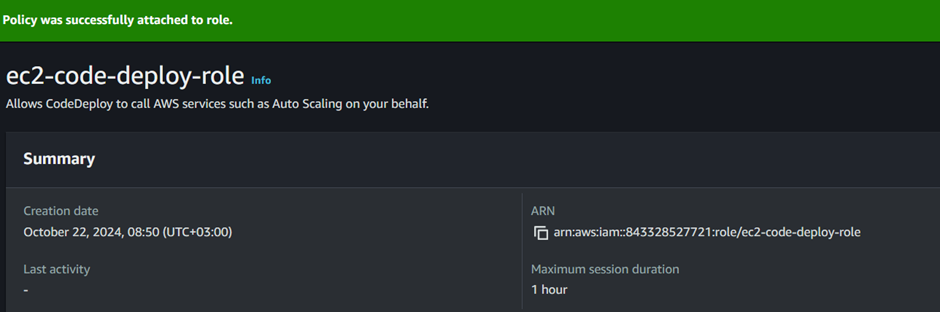
Attach the Role to Your EC2 Instance:
Once your role is created, attach it to your EC2 instance.
Select your instance from the Instances dashboard click on the Actionsdrop-down menu, then navigate to the Security tab.
Click on Modify IAM Role to attach the newly created role to your instance.
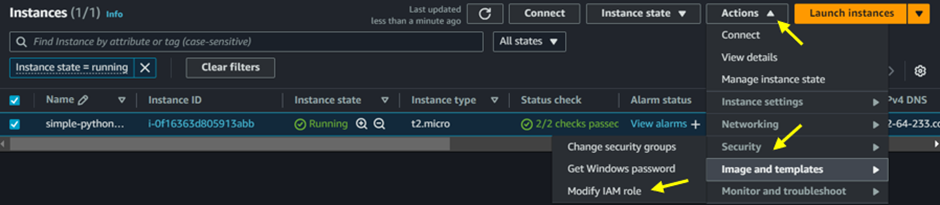
Choose the IAM role you created earlier from the dropdown menu then click Update Role to apply the changes.
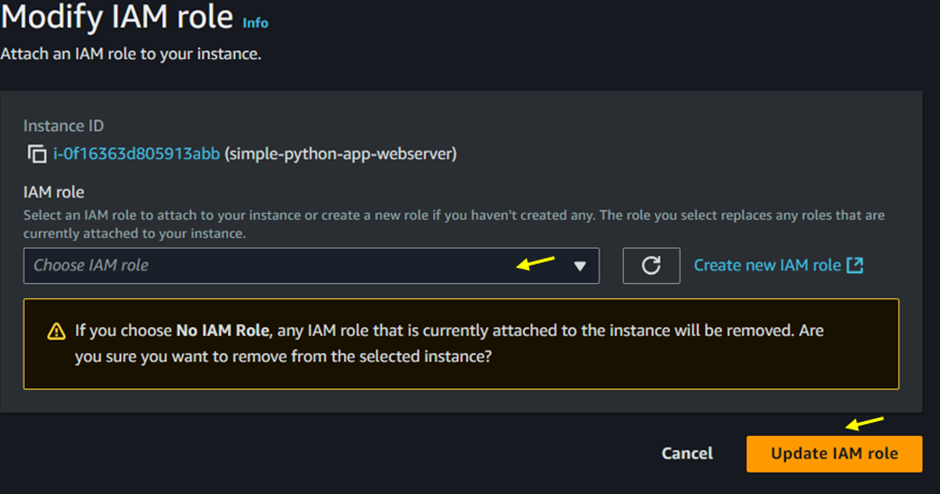
Installing the AWS CodeDeploy Agent
The AWS CodeDeploy agent is essential for enabling communication between your EC2 instance and the AWS CodeDeploy service. This agent manages deployments and ensures that application updates are orchestrated according to your deployment configurations.
Make sure to select the documentation that corresponds to the operating system of your EC2 instance. In my case, I am using Ubuntu. Use the following commands to install the codeDeploy agent on the Ubuntu instance.
# run system update
sudo apt update
sudo apt install ruby-full
sudo apt install wget
wget https://bucket-name.s3.region-identifier.amazonaws.com/latest/install
# your code should look like this
wget https://aws-codedeploy-us-east-1.amazonaws.com/latest/install
chmod +x ./install
sudo ./install auto
# automate the codeDeploy installation with the following shell script
#!/bin/bash
# Update package list
sudo apt update
# Install Ruby and wget if not already installed
sudo apt install -y ruby wget
# Navigate to the home directory
cd /home/ubuntu
# Download the CodeDeploy agent installer script for Ubuntu
wget https://aws-codedeploy-us-east-1.s3.us-east-1.amazonaws.com/latest/install
# Make the install script executable
chmod +x ./install
# Run the install script
sudo ./install auto
# Start the CodeDeploy agent service
sudo service codedeploy-agent start
# Check the status of the CodeDeploy agent
sudo service codedeploy-agent status
# Inform user of successful installation
echo "AWS CodeDeploy agent installed and started successfully."
# Save the script to a file, e.g., install_codedeploy.sh
# Make the script executable:
chmod +x install_codedeploy.sh
# Run the script
./install_codedeploy.sh
# This script installs the CodeDeploy agent and verifies that it's running. Make sure you replace the us-east-1 region in the S3 URL with your specific AWS region if you're not using us-east-1.
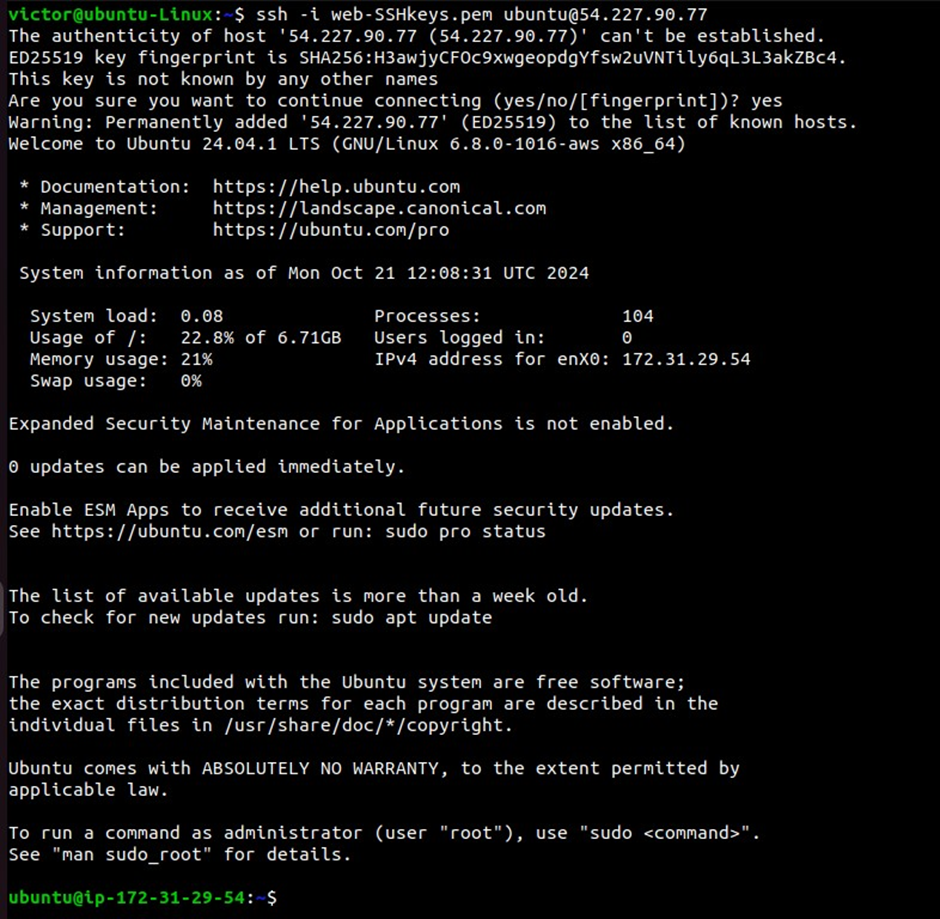
Verify the Installation:
After installing the AWS CodeDeploy agent, verify its installation by running the following command on your EC2 instance:
systemctl status codedeploy-agent
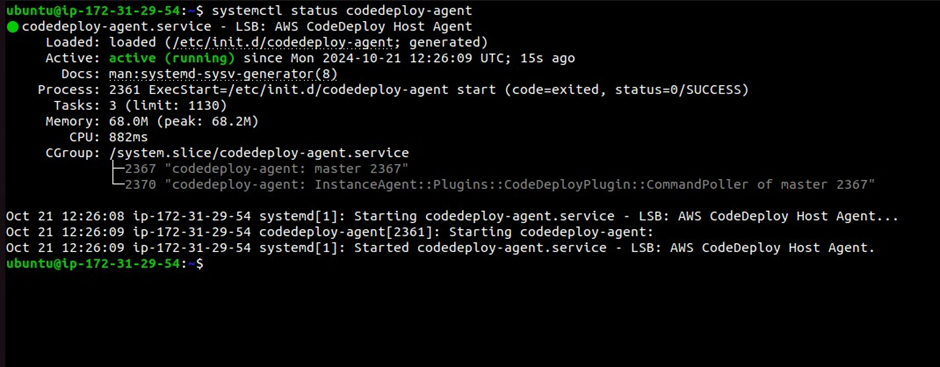
Install Docker:
Ensure that Docker is installed on your EC2 instance to use Docker commands later in the project. Use this shell script to install docker.
# make a file install_docker.sh
# using your favourite editor, paste in this code.
#!/bin/bash
# Update package list and install prerequisites
sudo apt update
sudo apt install -y apt-transport-https ca-certificates curl software-properties-common
# Add Docker’s official GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
# Add Docker's stable repository
echo "deb [arch=$(dpkg --print-architecture) signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
# Update package list to include Docker packages
sudo apt update
# Install Docker
sudo apt install -y docker-ce docker-ce-cli containerd.io
# Add the current user to the docker group
sudo usermod -aG docker $USER
# Inform user to log out and log back in for the group changes to take effect
echo "Docker installed and user added to docker group. Please log out and log back in to apply group changes."
# Make the script executable
chmod +x install_docker.sh
# Run the script
./install_docker.sh
# log out the you log back in.
Configure AWS CodeDeploy Application
To configure AWS CodeDeploy for automating application deployments across your AWS infrastructure, you need to first create an application. In the search bar, type CodeDeploy, then select CodeDeploy from the services list.
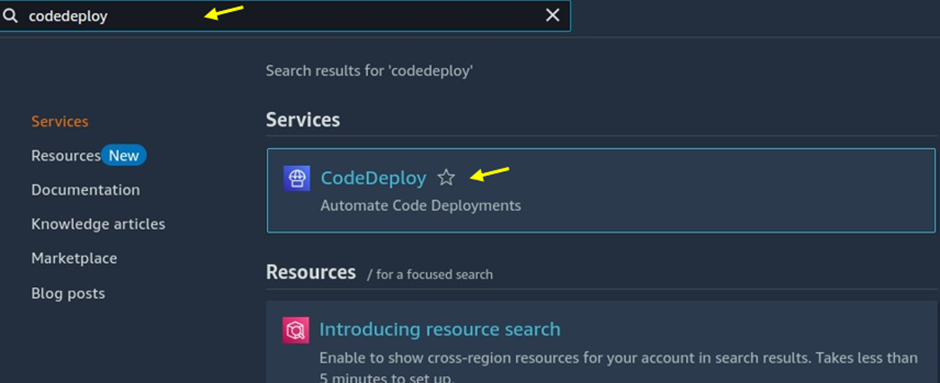
In the left-side menu, select Applications then click on the Create application button.
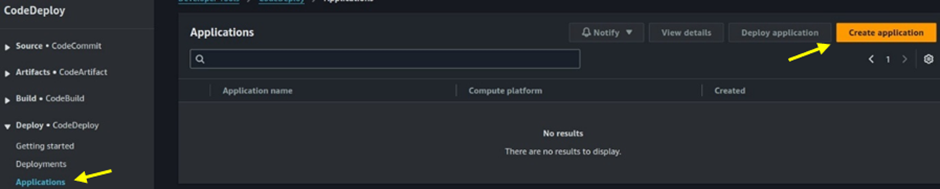
Enter the necessary details for your application then for the Compute platform, select EC2/On-premises. Click on Create application.
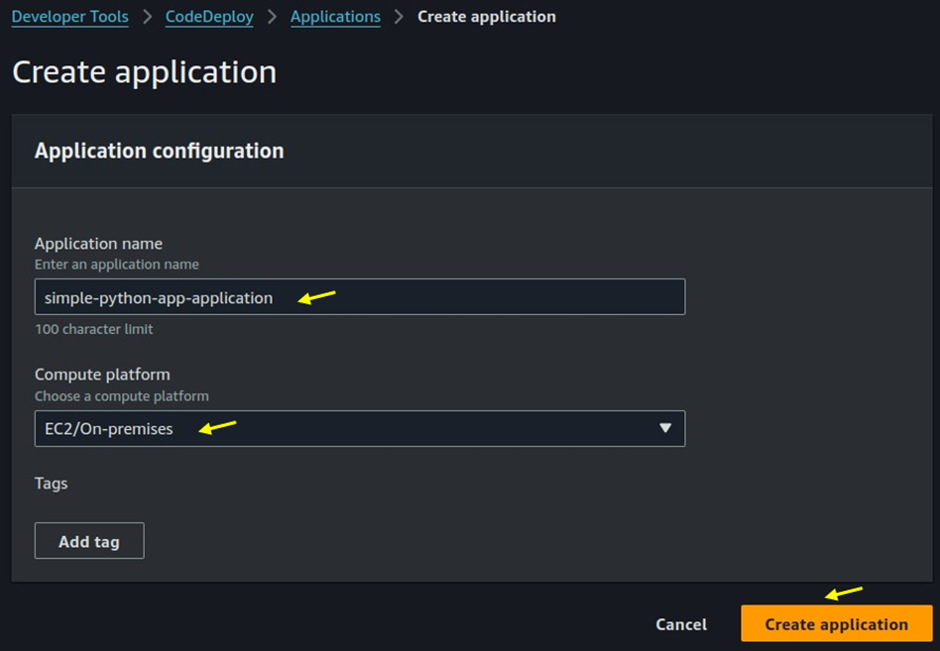
After creating your application, the next step is to create a deployment group. This is where you will select the EC2 instances for deployment.
Click on the application you just created, then navigate to the Deployments tab then click on Create Deployment Group.
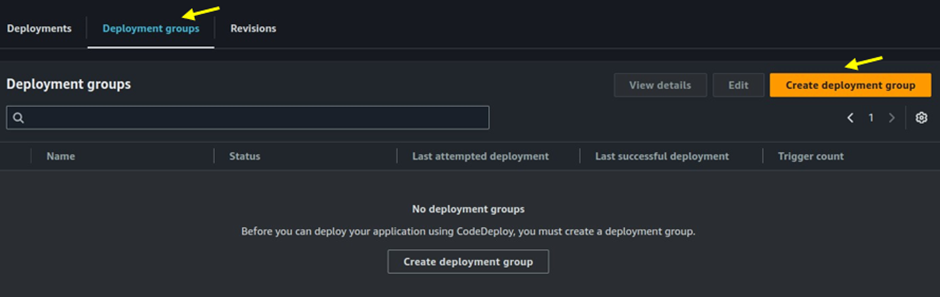
In the deployment group console, fill in the Deployment group name then for the Service role, select the IAM role you created earlier.
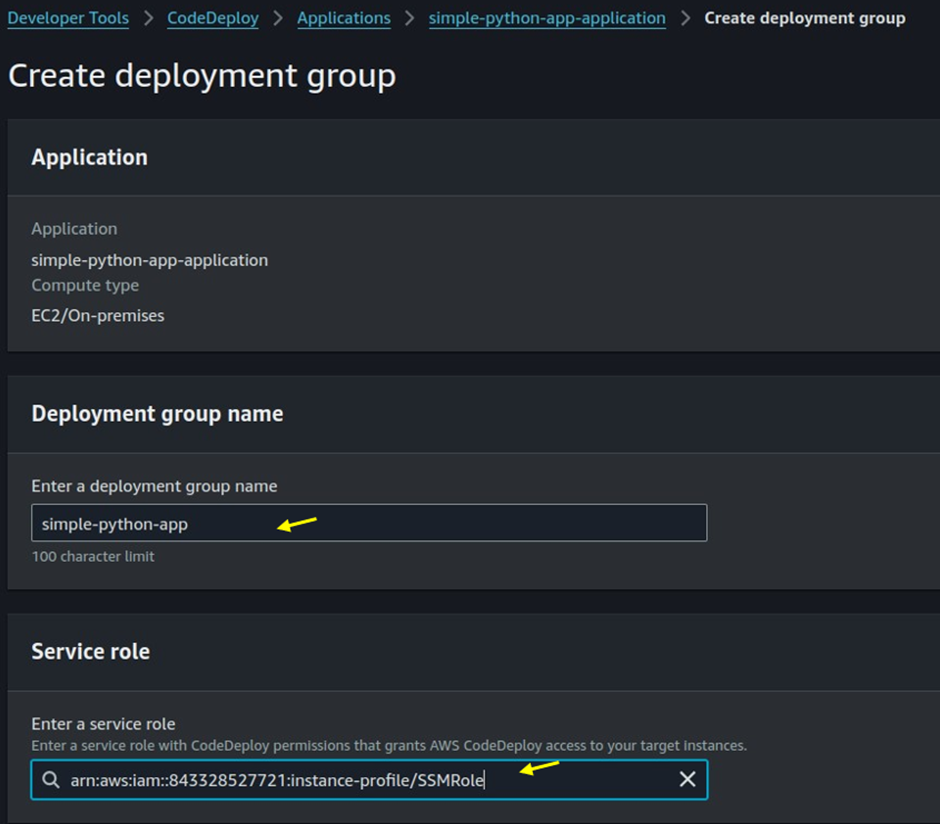
Under Environment configuration, select Amazon EC2 instances then in Target group 1, enter the tag of the instance you created. If you enter the correct tag, you should see one unique matched instance displayed.
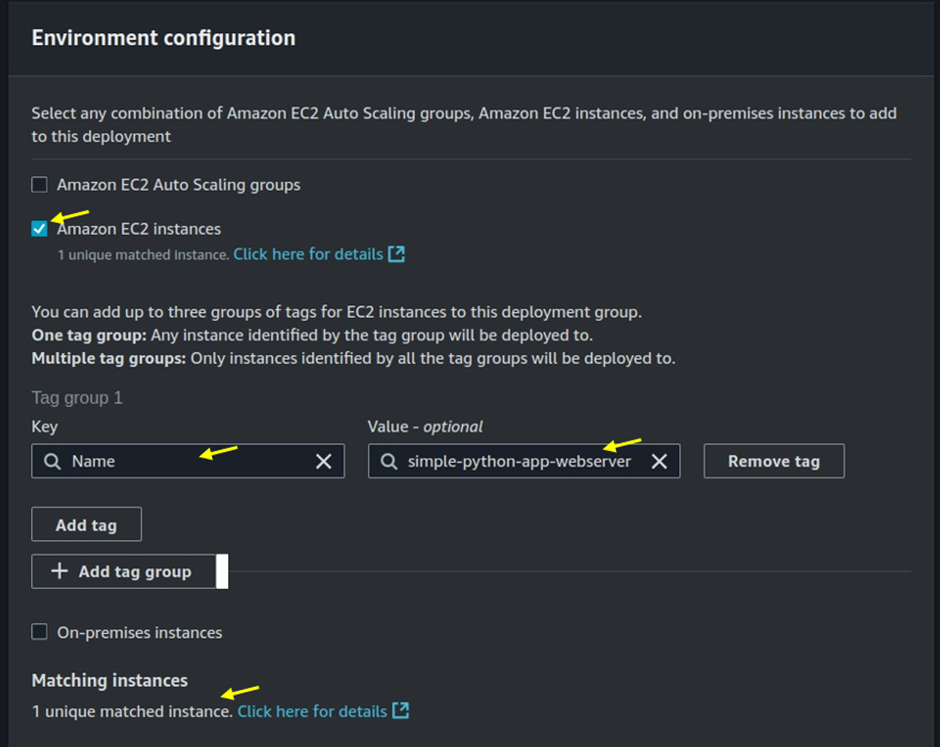
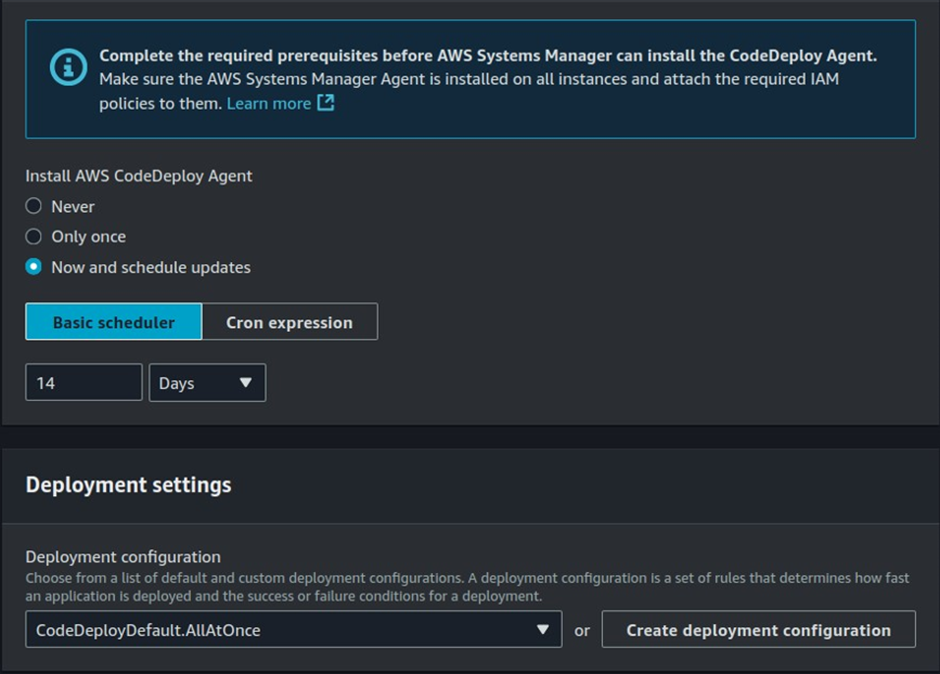
Proceed with the default CodeDeploysettings. Since a load balancer is not needed, uncheck the Load balance checkbox then click on Create deployment group.
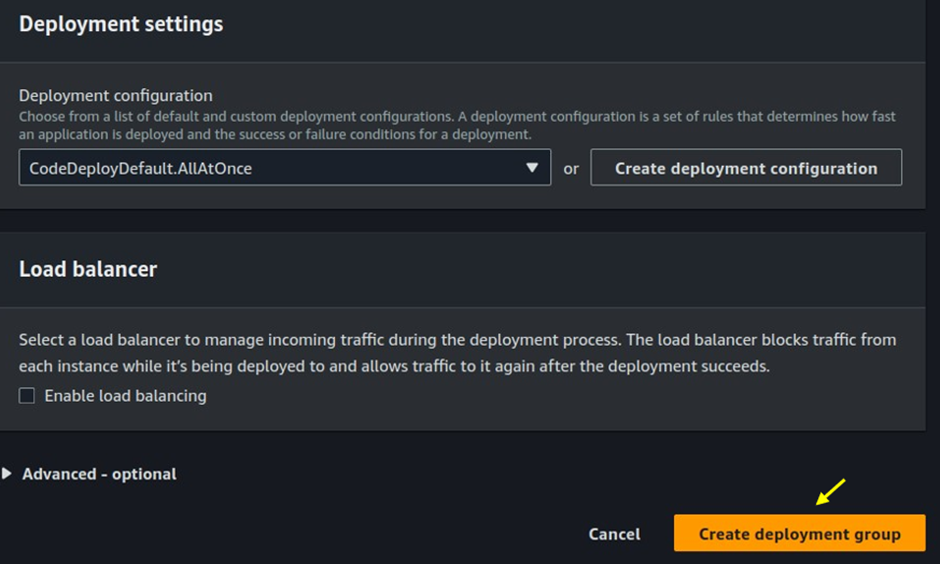
After creating the deployment group, the next step is to create a deployment for your application.
Click on the Deployments tab, then click on Create Deployments.
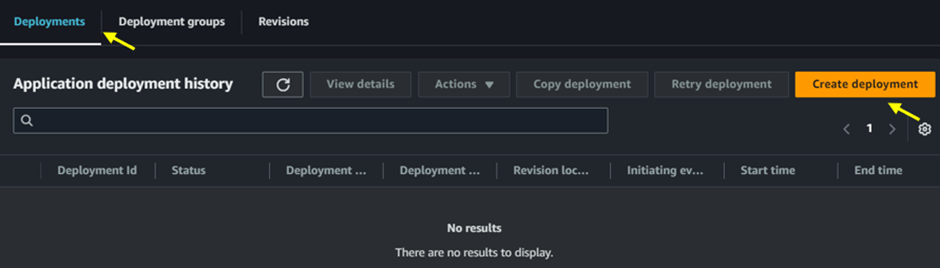
Configure Deployment Revision:
- Under Revision type, select My application is stored in GitHub.
- Enter your GitHub token name and provide your repository name along with the token ID.
- For Commit ID, while it’s not needed for the complete CI/CD process, since we’re verifying Continuous Deployment (CD) separately, you will need to supply it.
- Go to your GitHub repository, select one of the commits, and copy the Commit ID.
- Scroll down and click on Create deployment to finalize the process.
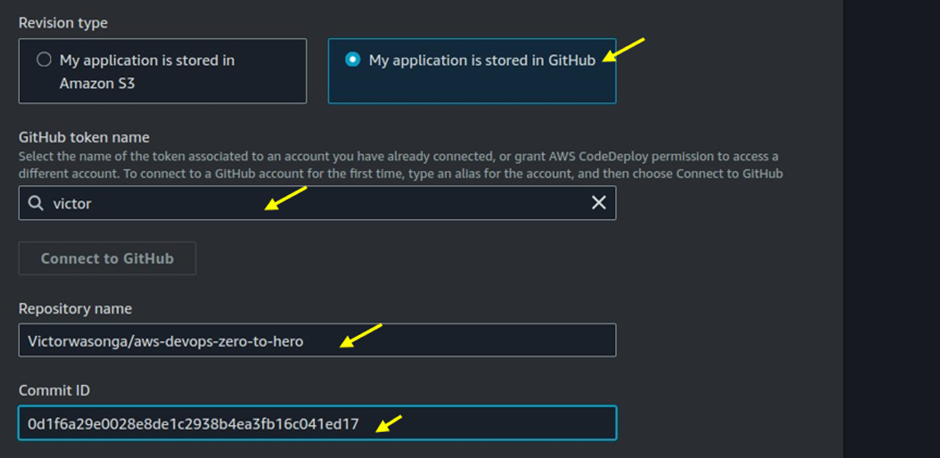
The deployment has been successfully created, and the status shows as Succeeded. This indicates that your application has been successfully deployed to your EC2 instance.
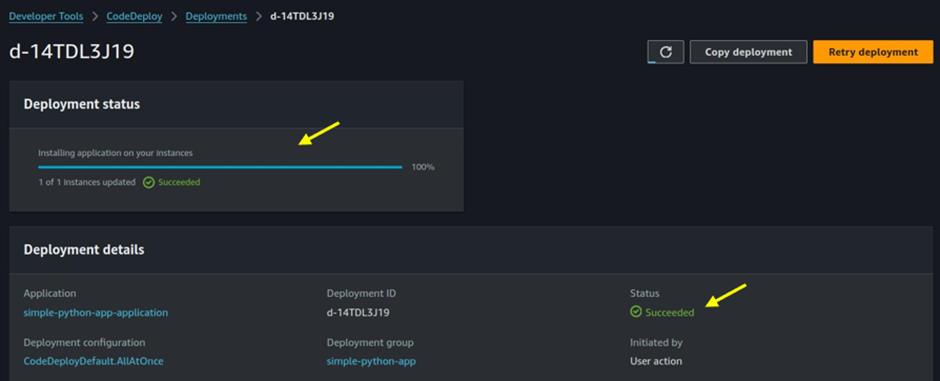
Integrate CodePipeline.
Next, we will integrate CodePipelineto to automatically trigger our deployment process whenever there is a code change in our GitHub repository.
In the search bar, type CodePipelineand select it from the services list.
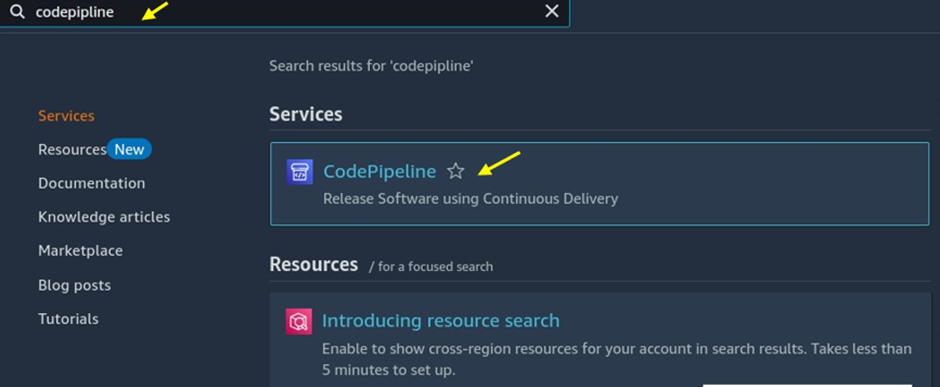
Edit CodePipeline: In our previous project, we had a CodePipeline integrated with our CodeBuild service. To add the CodeDeploy service, click the Edit button in the top right corner of the pipeline details page.
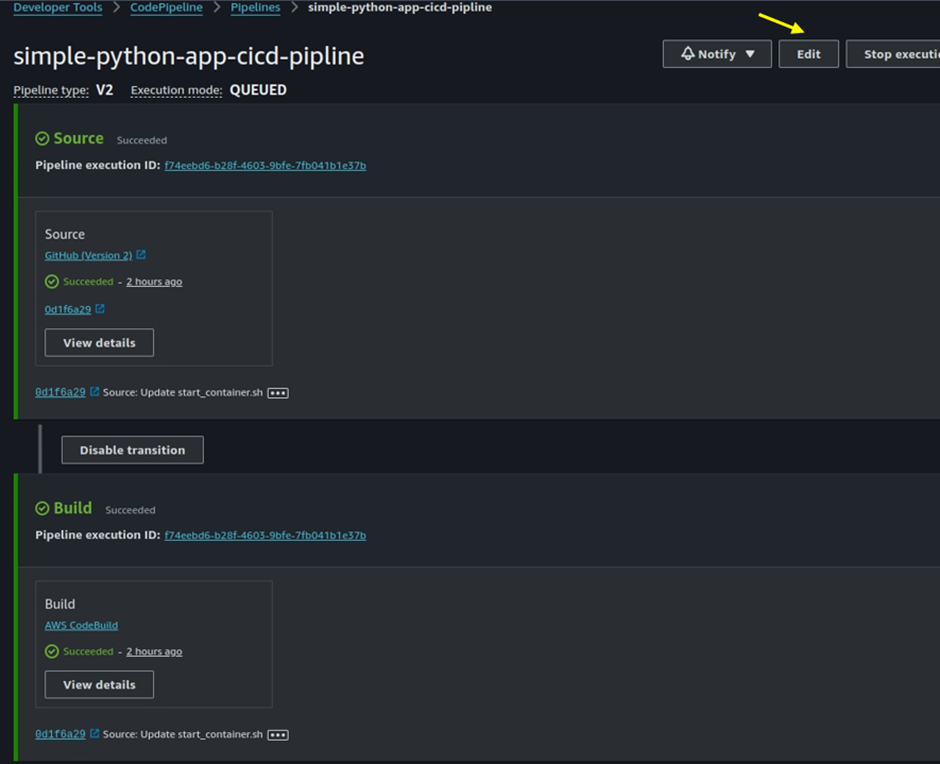
Add Deployment Stage: Scroll down to the Build stage.
Click on Add stage to insert a new stage for deployment.
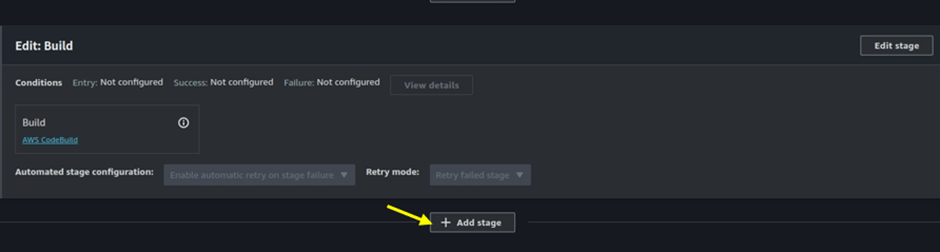
Enter a name for your new stage then click on Add stage.
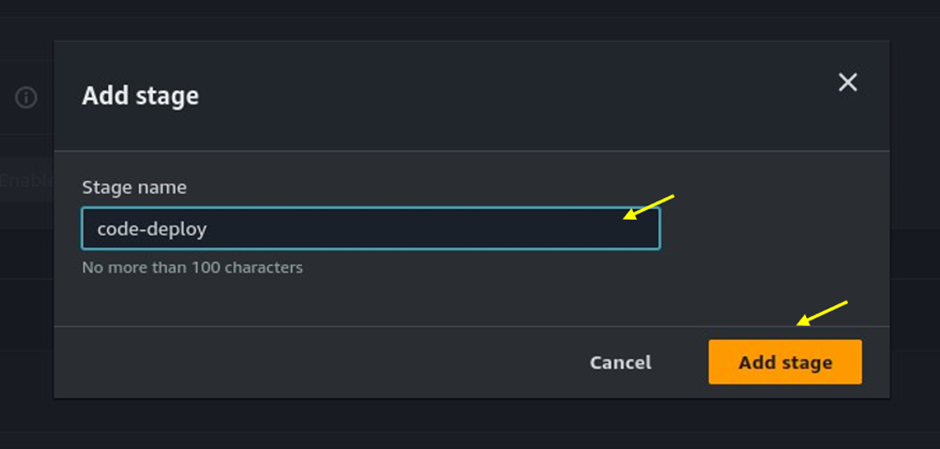
Add Action Group: Click on Add Action Group to define the actions that will be performed in this new stage.

Configure Action Group:
- Provide an Action name for this action group.
- In the Action provider dropdown, select CodeDeploy.
- Choose your preferred Region.
- For Input artifact, select the source artifact from the dropdown.
- Select your application name and Deployment group.
- Click on Done to complete the configuration.
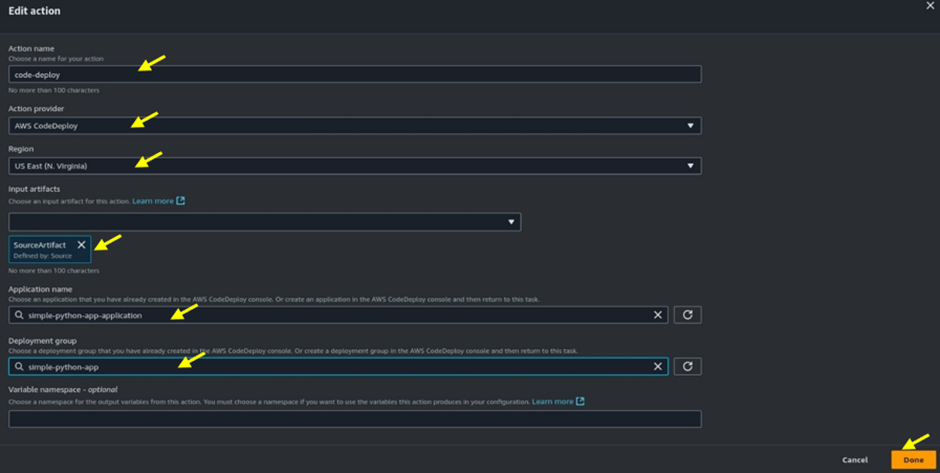
Navigate back to the top right corner of the pipeline page then click on Save to apply the changes you made to the pipeline.
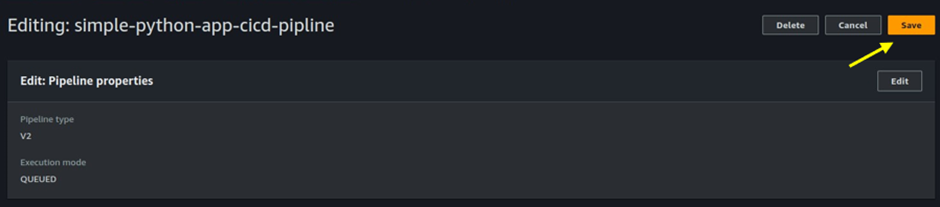
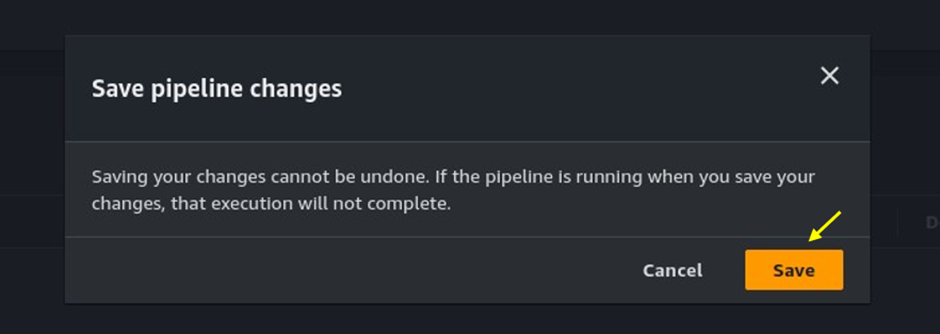
Trigger the Pipeline: You can either make changes to your code in your GitHub repository or click on Release change to manually start the pipeline.
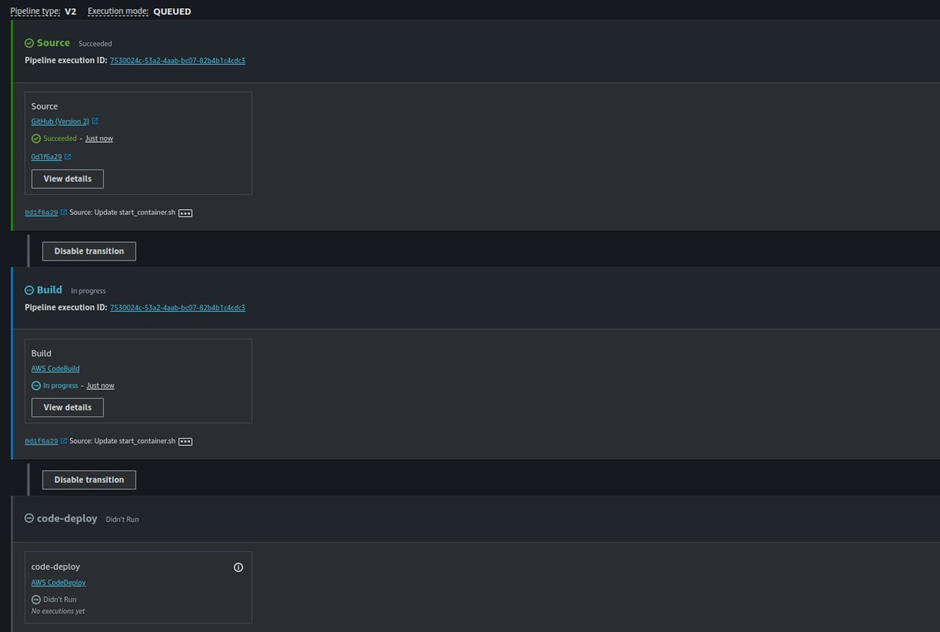
Our pipeline ran successfully, as indicated. Give yourself a round of applause for this achievement!
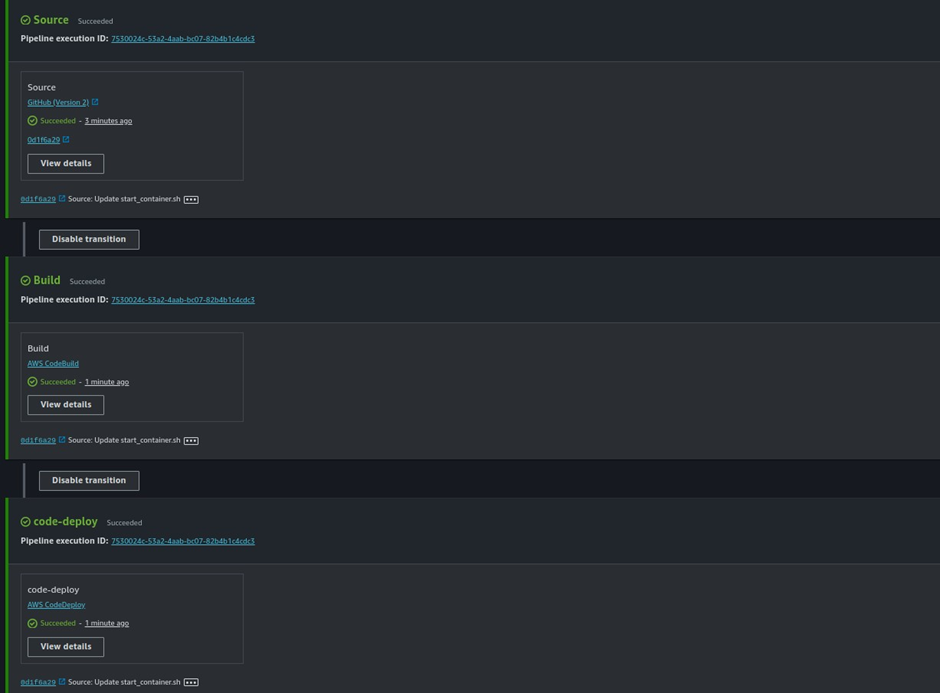
Conclusion
This project successfully implemented a Continuous Integration and Continuous Deployment (CI/CD) pipeline using AWS services, including CodePipeline, CodeBuild, and CodeDeploy. By automating the processes of building, testing, and deploying applications, we enhanced our development workflow and minimized the risk of human error. With automatic triggers for deployments based on code changes in our GitHub repository, we can quickly respond to updates. This project showcases the effectiveness of AWS tools in modern software development, promoting the benefits of CI/CD for improved software quality and faster delivery cycles.
Feel free to refer to this guide for setting up similar projects, and explore the benefits of continuous delivery using AWS CodeDeploy.
For more of my blog posts, you can click on this button.
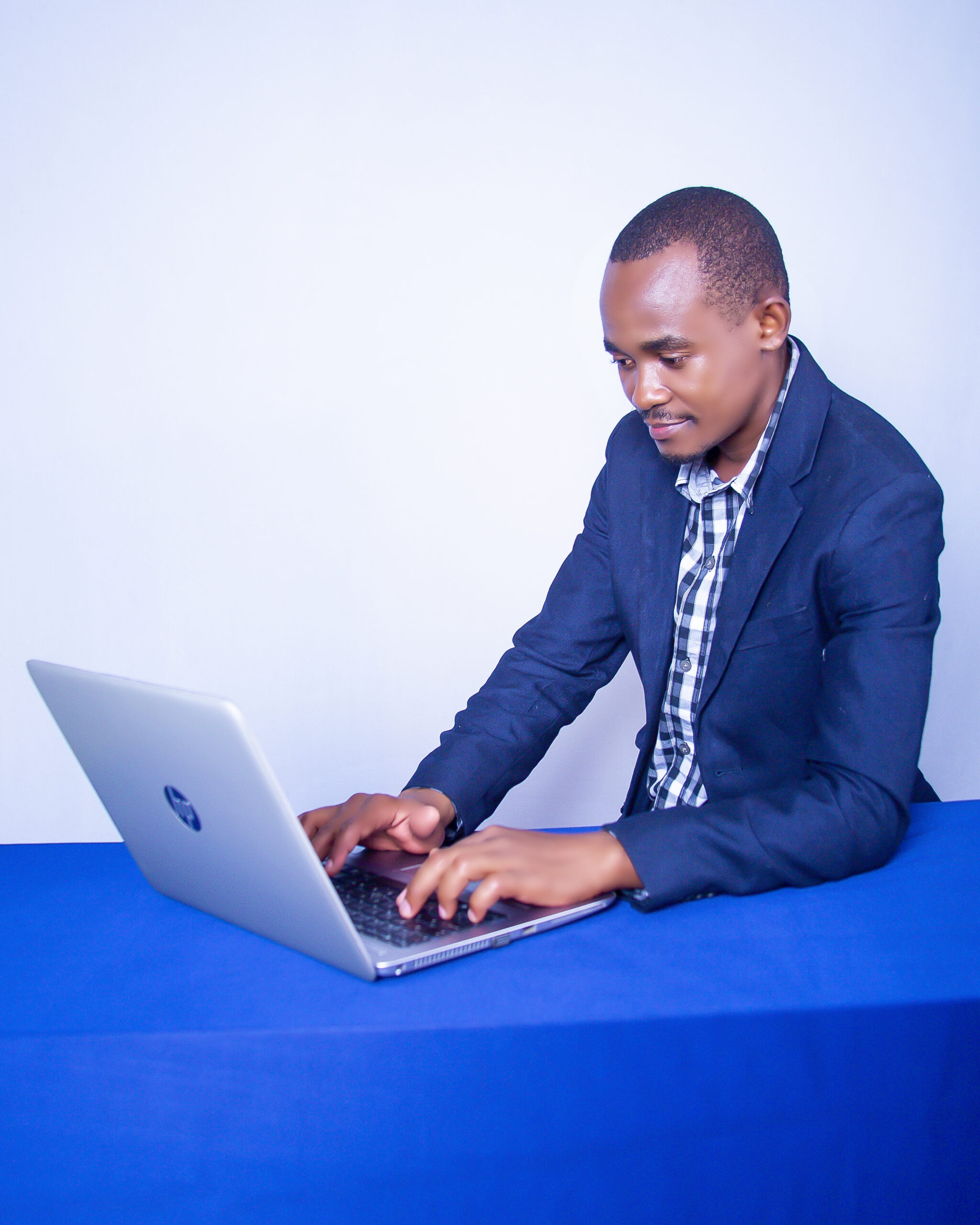
A skilled Cloud DevOps Engineer and Solutions Architect specializing in infrastructure provisioning and automation, with a focus on building scalable, fault-tolerant, and secure cloud environments.